How to Write an Add-on for Google Docs
You have seen examples of some really useful add-ons for Google Docs but wouldn’t it be great if you could write your own add-on, one that adds new features to your Google Docs, one that makes you a rock star among the millions of Google Docs users.
Well, it ain’t that difficult. If you know some HTML, CSS and JavaScript, you can create a Google Docs add-on.
Create a Google Add-on for Docs & Sheets
This step-by-step tutorial (download) will walk you through the process of creating your own add-on for Google Docs. The add-on used in the demo lets you insert a image of any address on Google Maps inside a Google Document without requiring any screen capture software.
Ok, lets’s get going.
Step 1. Open a new document inside Google Drive and choose Tools -> Script Editor. This is the Apps Script IDE where we’ll write the code for the add-on.
Step 2. Choose File -> New HTML to create a new HTML file inside the Script Editor and name your file as googlemaps.html (or anything you like).
Step 3. Copy-paste the following code in the HTML file and save your changes. This is the code that will be used to render the sidebar in your Google Documents.
<!-- Use this CSS stylesheet to ensure that add-ons styling
matches the default Google Docs styles -->
<link href="https://ssl.gstatic.com/docs/script/css/add-ons.css" rel="stylesheet" />
<!-- The sidebar will have a input box and the search button -->
<div class="sidebar">
<!-- The search box for Google Maps -->
<div class="form-group block">
<input type="text" id="search" placeholder="Enter address.. " />
<button class="blue" id="load_maps">Search Google Maps</button>
</div>
<!-- The container for the Google Maps static image -->
<div id="maps"></div>
</div>
<!-- Load the jQuery library from the Google CDN -->
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<script>
// Attach click handlers after the Sidebar has loaded in Google Docs
$(function () {
// Use Static Maps to generate an image of the address entered by the user
$('#load_maps').click(function () {
var mapURL =
'https://maps.googleapis.com/maps/api/staticmap?center=' +
encodeURIComponent($('#search').val()) +
'&zoom=14&size=200x400&sensor=false';
$('#maps').html('<img src=" ' + mapURL + ' "/>');
});
// If the user presses the Enter key in the search box, perform a search
$('#search').keyup(function (e) {
if (e.keyCode === 13) {
$('#load_maps').click();
}
});
// When a user clicks the thumbnail image in the sidebar, call
// insertGoogleMap to insert the maps image in the current document
$('#maps').click(function () {
google.script.run.insertGoogleMap($('#search').val());
});
});
</script>
Step 4. Next we will write the server side JavaScript (Google Script) that will actually render the sidebar and insert Google Maps images in the document.
/* What should the add-on do after it is installed */
function onInstall() {
onOpen();
}
/* What should the add-on do when a document is opened */
function onOpen() {
DocumentApp.getUi()
.createAddonMenu() // Add a new option in the Google Docs Add-ons Menu
.addItem("Google Maps", "showSidebar")
.addToUi(); // Run the showSidebar function when someone clicks the menu
}
/* Show a 300px sidebar with the HTML from googlemaps.html */
function showSidebar() {
var html = HtmlService.createTemplateFromFile("googlemaps")
.evaluate()
.setTitle("Google Maps - Search"); // The title shows in the sidebar
DocumentApp.getUi().showSidebar(html);
}
/* This Google Script function does all the magic. */
function insertGoogleMap(e) {
var map = Maps.newStaticMap()
.setSize(800, 600) // Insert a Google Map 800x600 px
.setZoom(15)
.setCenter(e); // e contains the address entered by the user
DocumentApp.getActiveDocument()
.getCursor() // Find the location of the cursor in the document
.insertInlineImage(map.getBlob()); // insert the image at the cursor
}
Save your changes and then choose onOpen from the Run menu inside the Script editor. Authorize the script and switch to your Google Document.
You’ll see a new Google Maps option under the Add-ons menu. Select the menu item and you’ll be able to insert maps images inside your Google Documents without using any screen capture software.
Share your Google Add-ons with other Google Docs users
Now that your first Google add-on is ready, you may want to distribute it to other users of Google Docs. The easiest option would be that you share your document with public and set the permission as Anyone can view. Now anyone can create a copy of your document in their own Google Drive and use your add-on.
Google Add-ons can also be published to the Chrome store, the process is similar to publishing Chrome extensions, but this isn’t available to all Google developers yet.
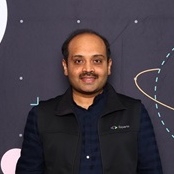
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory