Search Books with Goodreads API and Google Apps Script
The Goodreads API helps you query the entire database of books on the Goodreads website. You can find the ratings of books, fetch book reviews, search books by author or even publish your own reviews. This example shows how to connect to the GoodReads website through Google Apps Script, find books by title, parse the XML results as JSON and write the results in a Google Spreadsheet.
You can also extend the code to insert the thumbnail of the book image in a spreadsheet cell using the IMAGE function.
To get started, go to the Goodreads.com account and create a key. All Rest API methods will require you to register for a developer key.
Goodreads will return the response in XML format (see below) and we can use the XML service of Google Apps Script to easily parse this XML response.
Here’s the complete example. Remember to replace the API key with your own.
function GoodReads() {
var search = 'Taj Mahal';
var books = searchBooks_(search);
// Write Data to Google Spreadsheet.
var sheet = SpreadsheetApp.getActiveSheet();
books.forEach(function (book) {
sheet.appendRow([book.title, book.author, book.rating, book.url]);
});
}
function searchBooks_(query) {
var baseUrl = 'https://www.goodreads.com/book/show/',
apiUrl = 'https://www.goodreads.com/search/index.xml',
apiKey = 'ctrlq.org',
searchResults = [],
payload = {
q: query,
key: apiKey,
},
params = {
method: 'GET',
payload: payload,
muteHttpExceptions: true,
};
var response = UrlFetchApp.fetch(apiUrl, params);
// API Connection Successful
if (response.getResponseCode() === 200) {
// Parse XML Response
var xml = XmlService.parse(response.getContentText());
var results = xml.getRootElement().getChildren('search')[0];
// Save the result in JSON format
results
.getChild('results')
.getChildren()
.forEach(function (result) {
result.getChildren('best_book').forEach(function (book) {
searchResults.push({
title: book.getChild('title').getText(),
author: book.getChild('author').getChild('name').getText(),
thumbnail: book.getChild('image_url').getText(),
rating: result.getChild('average_rating').getText(),
url: baseUrl + result.getChild('id').getText(),
});
});
});
}
return searchResults;
}
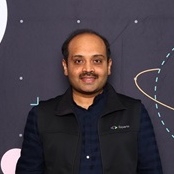
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory