Send SMS with Google Scripts and Twilio
The Twilio service helps you send SMS and MMS programmatically. They have a REST API that you can call through Google Apps Script and send SMS text messages from your Google Apps based projects. For instance, you can get a text notification on a mobile phone when a Google Form is submitted. Or you can send short text messages to multiple people from a Google Spreadsheet similar to Mail Merge.
To get started, you need to register for an account at Twilio (they have trial accounts too) and send text any phone number in the world via Google Scripts. You will use your Twilio account SID as the username and your auth token as the password for HTTP Basic authentication.
/*
Send SMS via #AppsScript
=========================
Written by Amit Agarwal
Website: ctrlq.org
Email: amit@labol.org
Twitter: @labnol
*/
function sendSMS(toNumber, fromNumber, smsText) {
if (smsText.length > 160) {
Logger.log('The text should be limited to 160 characters');
return;
}
var accountSID = 'ctrlq.sid';
var authToken = 'ctrlq.token';
var url = 'https://api.twilio.com/2010-04-01/Accounts/' + accountSID + '/Messages.json';
var options = {
method: 'POST',
headers: {
Authorization: 'Basic ' + Utilities.base64Encode(accountSID + ':' + authToken),
},
payload: {
From: fromNumber,
To: toNumber,
Body: smsText,
},
muteHttpExceptions: true,
};
var response = JSON.parse(UrlFetchApp.fetch(url, options).getContentText());
if (response.hasOwnProperty('sid')) {
Logger.log('Message sent successfully.');
}
Utilities.sleep(1000);
}
Sending SMS with Twilio - Notes
- The recipient’s phone number should be formatted with a ’+’ and always include the country code e.g., +16175551212 (E.164 format). 2. The SMS body should be less than 160 characters else Twillo would split the text into multiple messages. 3. The sender’s phone number should be a valid Twilio phone number. You cannot put just any mobile phone number to prevent spoofing.
It is important to add sleep between consecutive SMS sending calls as Twilio will only send out messages at a rate of one message per phone number per second.
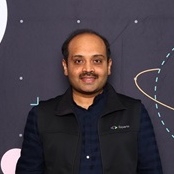
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory