Validate an Email Address with PHP
Before sending email via SMTP, the client computer initiates a transaction with the SMTP email server that is listening for commands on TCP port 25. The SMTP command HELO initiates a transaction with the mail server and identifies itself, MAIL FROM and RCTP specify the sender and recipients respectively while QUIT will close the conversation. If the mail server returns the status as 250, that means the email address is validate and exists.
@hbattat has written a wrapper PHP library that can be used to determine if an email address is real or not. You specify the sender’s email, the recipient’s email and connect to the mail server to know whether that email exists on the domain or not. Email verification can be done using Windows Telnet as well.
/* Credit: https://github.com/hbattat/verifyEmail */
function verifyEmail($toemail, $fromemail, $getdetails = false)
{
// Get the domain of the email recipient
$email_arr = explode('@', $toemail);
$domain = array_slice($email_arr, -1);
$domain = $domain[0];
// Trim [ and ] from beginning and end of domain string, respectively
$domain = ltrim($domain, '[');
$domain = rtrim($domain, ']');
if ('IPv6:' == substr($domain, 0, strlen('IPv6:'))) {
$domain = substr($domain, strlen('IPv6') + 1);
}
$mxhosts = array();
// Check if the domain has an IP address assigned to it
if (filter_var($domain, FILTER_VALIDATE_IP)) {
$mx_ip = $domain;
} else {
// If no IP assigned, get the MX records for the host name
getmxrr($domain, $mxhosts, $mxweight);
}
if (!empty($mxhosts)) {
$mx_ip = $mxhosts[array_search(min($mxweight), $mxhosts)];
} else {
// If MX records not found, get the A DNS records for the host
if (filter_var($domain, FILTER_VALIDATE_IP, FILTER_FLAG_IPV4)) {
$record_a = dns_get_record($domain, DNS_A);
// else get the AAAA IPv6 address record
} elseif (filter_var($domain, FILTER_VALIDATE_IP, FILTER_FLAG_IPV6)) {
$record_a = dns_get_record($domain, DNS_AAAA);
}
if (!empty($record_a)) {
$mx_ip = $record_a[0]['ip'];
} else {
// Exit the program if no MX records are found for the domain host
$result = 'invalid';
$details .= 'No suitable MX records found.';
return ((true == $getdetails) ? array($result, $details) : $result);
}
}
// Open a socket connection with the hostname, smtp port 25
$connect = @fsockopen($mx_ip, 25);
if ($connect) {
// Initiate the Mail Sending SMTP transaction
if (preg_match('/^220/i', $out = fgets($connect, 1024))) {
// Send the HELO command to the SMTP server
fputs($connect, "HELO $mx_iprn");
$out = fgets($connect, 1024);
$details .= $out."n";
// Send an SMTP Mail command from the sender's email address
fputs($connect, "MAIL FROM: <$fromemail>rn");
$from = fgets($connect, 1024);
$details .= $from."n";
// Send the SCPT command with the recepient's email address
fputs($connect, "RCPT TO: <$toemail>rn");
$to = fgets($connect, 1024);
$details .= $to."n";
// Close the socket connection with QUIT command to the SMTP server
fputs($connect, 'QUIT');
fclose($connect);
// The expected response is 250 if the email is valid
if (!preg_match('/^250/i', $from) || !preg_match('/^250/i', $to)) {
$result = 'invalid';
} else {
$result = 'valid';
}
}
} else {
$result = 'invalid';
$details .= 'Could not connect to server';
}
if ($getdetails) {
return array($result, $details);
} else {
return $result;
}
}
SMTP also provides the VRFY command to query when the specified user exists on the host or not but it is often ignored by mail servers.
Also see: Find Person by Email Address
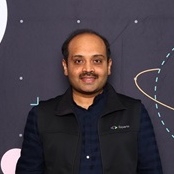
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory