Convert XML to JSON with Apps Script
The XMLService class of Google Apps Scripts lets you parse and navigate the nodes of any XML file. You can also convert the XML to JSON and access the XML nodes though the simpler dot notation.
Unlike the deprecated XML class, the new XMLService class doesn’t allow lenient parsing and hence may not be parse the HTML DOM structure since it may not be well formed.
/* Source: https://gist.github.com/erickoledadevrel/6b1e9e2796e3c21f669f */
/**
* Converts an XML string to a JSON object, using logic similar to the
* sunset method Xml.parse().
* @param {string} xml The XML to parse.
* @returns {Object} The parsed XML.
*/
function XML_to_JSON(xml) {
var doc = XmlService.parse(xml);
var result = {};
var root = doc.getRootElement();
result[root.getName()] = elementToJSON(root);
return result;
}
/**
* Converts an XmlService element to a JSON object, using logic similar to
* the sunset method Xml.parse().
* @param {XmlService.Element} element The element to parse.
* @returns {Object} The parsed element.
*/
function elementToJSON(element) {
var result = {};
// Attributes.
element.getAttributes().forEach(function (attribute) {
result[attribute.getName()] = attribute.getValue();
});
// Child elements.
element.getChildren().forEach(function (child) {
var key = child.getName();
var value = elementToJSON(child);
if (result[key]) {
if (!(result[key] instanceof Array)) {
result[key] = [result[key]];
}
result[key].push(value);
} else {
result[key] = value;
}
});
// Text content.
if (element.getText()) {
result['Text'] = element.getText();
}
return result;
}
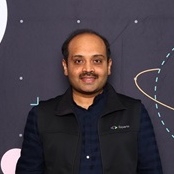
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory