Google Apps Script for Website Monitoring
This Google Apps Script will turn Google Docs into a website uptime monitor.
/** Monitor your Site's Uptime **/
function isMySiteDown() {
// Get the URL of the Website to monitor
var url = SpreadsheetApp.getActiveSheet().getRange('E3').getValue();
// HTTP Response Code of the last server request
if (!ScriptProperties.getProperty('status')) {
ScriptProperties.setProperty('status', 200);
}
var response, error;
try {
// Fetch the web page using UrlFetchApp
response = UrlFetchApp.fetch(url);
} catch (error) {
insertData(error, -1, 'Website down');
return;
}
var code = response.getResponseCode();
// code = 200 means the fetch request was successful
if (code == 200) insertData('Up', code, 'Website up');
else insertData(response.getContent()[0], code, 'Website down');
}
function insertData(error, code, msg) {
// Ignore if the error message is logged already
if (ScriptProperties.getProperty('status') == code) return;
// Log the server error in a Google Sheet
var sheet = SpreadsheetApp.getActiveSheet();
var email = sheet.getRange('E5').getValue();
var row = sheet.getLastRow() + 1;
sheet.getRange(row, 1).setValue(new Date());
sheet.getRange(row, 2).setValue(error);
sheet.getRange(row, 3).setValue(code);
// Send an email alert for the downtime
ScriptProperties.setProperty('status', code);
MailApp.sendEmail(email, msg, error);
}
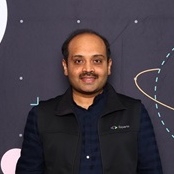
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory