The 43 Folders System to Organize your Gmail
43 Folders is a ticker file system that is used to file time-sensitive documents, appointments and emails in such a manner that they are labeled according to a date on which the document needs to be acted upon. There are 12 folders for every month and 31 folders for every day and hence the name 43 folders. This useful Google Script lets you organize your Gmail messages around the concept of 43 folders. It was originally published by Facundo Bromberg but the domain hosting the script had gone offline. Luckily, Google Cache had a copy.
I present here an adaptation of Google’s snooze script that works under the 43folders methodology. The original script posted by Corey Goldfeder allows you to snooze an email a certain amount of days by labeling it with a label indicating the number of days to snooze it, e.g., Snooze1day. After archiving, the email would re-appear in your inbox after 1 day. Inspired by this script I created the 43folders script that implements a tickler file with the 43 folders methodology to postpone tasks (for a detailed explanation of the 43 folder methodology see for example here).
The 43 methodology considers 43 folders labeled 01 through 31 and JAN,FEB,MAR,…,DEC, i.e., 31+12 = 43 folders. The idea of the 43 folders is to give 1 day granularity for the next 31 days, otherwise postpone it to the beginning of a month. For instance, if today is August 22nd and I put something in folder 24, I am postponing it to the 24th of August, if I put it in folder 04 I am postponing it until September 4th. If I need to postpone something to September 22nd onwards I put it in a month folder, for instance, if I put it in folder FEB, I am postponing it to next February 2012. The idea is that at the beginning of each month I should re-organize whatever is in the month’s folder.
How does 43folders for Gmail work
- There are 43 labels, one per folder, i.e., 01-31 and JAN-DEC.
- To “put” an email in a folder simply label it and archive.
- If you labeled it with a day, e.g. 24, the email will automatically show back in your inbox on that day, i.e., the 24th. If you labeled it with a month, e.g. FEB, it will show back in your inbox the first day of that month, i.e., February 1st 2012.
- The script has two shortcuts labels: “atomorrow” and “a_nextweek” (the a’s to have them above all others in gmail). These labels works as expected, an email labeled a tomorrow will show back in your email, well, tomorrow; and an email labeled next_week will show back in your inbox next Monday.
How to Setup 43 Folders for Gmail
Go to Google Docs and create a new spreadsheet, then choose “Script Editor” from the “Tools” menu. Paste in the following code:
//-----------------------------------------------
// SETTINGS:
//
// 1. Choose your prefered base and unsnoozed labels.
// 2. Choose whether you want the unsnoozed emails to be marked unread and
// labeled UNSNOOZED_LABEL
// 3. Set a daily trigger for method process().
//-----------------------------------------------
var MARK_UNREAD = true;
var MARK_UNSNOOZED = true;
var UNSNOOZED_LABEL = 'A/UNSNZD';
var BASE_LABEL = 'C/z43f';
//-----------------------------------------------
//-----------------------------------------------
//-----------------------------------------------
var TOMORROW_LABEL = BASE_LABEL + '/a_tomorrow';
var NEXTWEEK_LABEL = BASE_LABEL + '/a_nextWeek';
var SNOOZED_LABEL = 'A/SNZD';
var TODAY_LABEL = BASE_LABEL + '/today';
var THISWEEK_LABEL = BASE_LABEL + '/thisWeek';
var THISMONTH_LABEL = BASE_LABEL + '/thisMonth';
//-----------------------------------------------
function getDayLabelName(i) {
if (i <= 9) return BASE_LABEL + '/0' + i;
else return BASE_LABEL + '/' + i;
}
//-----------------------------------------------
function getMonthLabelName(i) {
if (i == 1) return BASE_LABEL + '/JAN';
if (i == 2) return BASE_LABEL + '/FEB';
if (i == 3) return BASE_LABEL + '/MAR';
if (i == 4) return BASE_LABEL + '/APR';
if (i == 5) return BASE_LABEL + '/MAY';
if (i == 6) return BASE_LABEL + '/JUN';
if (i == 7) return BASE_LABEL + '/JUL';
if (i == 8) return BASE_LABEL + '/AUG';
if (i == 9) return BASE_LABEL + '/SEP';
if (i == 10) return BASE_LABEL + '/OCT';
if (i == 11) return BASE_LABEL + '/NOV';
if (i == 12) return BASE_LABEL + '/DEC';
}
//-----------------------------------------------
function setup() {
// Create the labels we’ll need for snoozing
GmailApp.createLabel(BASE_LABEL);
GmailApp.createLabel(TOMORROW_LABEL);
GmailApp.createLabel(NEXTWEEK_LABEL);
GmailApp.createLabel(SNOOZED_LABEL);
GmailApp.createLabel(UNSNOOZED_LABEL);
for (var i = 1; i <= 31; ++i) {
GmailApp.createLabel(getDayLabelName(i));
}
for (var i = 1; i <= 12; ++i) {
GmailApp.createLabel(getMonthLabelName(i));
}
GmailApp.createLabel(TODAY_LABEL);
GmailApp.createLabel(THISMONTH_LABEL);
}
//-----------------------------------------------
function process() {
var today = new Date();
var weekday = today.getDay();
var monthday = today.getDate();
var month = today.getMonth() + 1;
var year = today.getYear();
moveToInbox(GmailApp.getUserLabelByName(getDayLabelName(monthday)), TODAY_LABEL);
moveToInbox(GmailApp.getUserLabelByName(TOMORROW_LABEL), TODAY_LABEL);
moveToInbox(GmailApp.getUserLabelByName(getMonthLabelName(month)), THISMONTH_LABEL);
if (weekday == 1) {
moveToInbox(GmailApp.getUserLabelByName(NEXTWEEK_LABEL), THISWEEK_LABEL);
}
}
//-----------------------------------------------
function moveToInbox(label, newLabelName) {
page = null;
// Get threads in "pages" of 100 at a time
while (!page || page.length == 100) {
page = label.getThreads(0, 100);
if (page.length > 0) {
GmailApp.moveThreadsToInbox(page);
if (MARK_UNREAD) {
GmailApp.markThreadsUnread(page);
}
//Mark with new label
//GmailApp.getUserLabelByName(newLabelName).addToThreads(page);
//Adds UNSNOOZED_LABEL
if (MARK_UNSNOOZED) {
GmailApp.getUserLabelByName(UNSNOOZED_LABEL).addToThreads(page);
}
// Removes label
label.removeFromThreads(page);
//Removes SNOOZED_LABEL in case it was added by maintenance (addBASE_LABEL script) or manually
GmailApp.getUserLabelByName(SNOOZED_LABEL).removeFromThreads(page);
}
}
}
//-----------------------------------------------
// Adds the label to all emails with some 43f sublabel. This is for easy hiding in multiple-inbox.
function addLABEL() {
var labelName = SNOOZED_LABEL;
addLabel(GmailApp.getUserLabelByName(NEXTWEEK_LABEL), labelName);
addLabel(GmailApp.getUserLabelByName(TOMORROW_LABEL), labelName);
for (var i = 1; i <= 31; ++i) {
addLabel(GmailApp.getUserLabelByName(getDayLabelName(i)), labelName);
}
for (var i = 1; i <= 12; ++i) {
addLabel(GmailApp.getUserLabelByName(getMonthLabelName(i)), labelName);
}
}
//-----------------------------------------------
function addLabel(label, newLabelName) {
page = null;
// Get threads in "pages" of 100 at a time
while (!page || page.length == 100) {
page = label.getThreads(0, 100);
if (page.length > 0) GmailApp.getUserLabelByName(newLabelName).addToThreads(page);
}
}
Then click the “Save” button and give it a name. In the dropdown labeled “Select a function to run.” choose “setup” and click the blue run arrow to the left of it. This will ask you to authorize the script, and will create the necessary labels in your Gmail. Then go to the “Triggers” menu and choose “current script’s triggers”. Click the link to set up a new trigger, choose the “process” function, a “time-driven” event, “day timer”, and then “midnight to 1am.” Click save and you’re done.
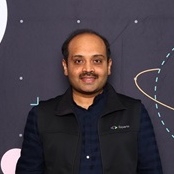
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory