How to Use Cron Expressions to Create Time Triggers in Apps Script
How to work with Cron Expressions inside Google Apps Script and setup time triggers for scheduling task at recurring intervals.
Cron is a scheduling tool that helps you run tasks at recurring intervals. You use a cron expression to specify the exact timing for your scheduled task. For example, if you want a schedule to run every week day at 8:30 pm, the cron expression would look like this:
30 20 * * 1-5
Cron Expressions
Here are some more practical examples to help you understand the cron expression.
Cron Expression | Description |
---|---|
0 0 * * * | every day at midnight |
0 */2 * * * | every 2 hours |
0 10 * * FRI,SAT | every Friday and Saturday at 10 am |
30 9 */15 * * | at 9:30 am on every 15th day |
0 0 1 */3 * | first day of every quarter |
Time Triggers in Google Apps Script
Google Apps Script supports time-driven triggers to help you run tasks in the background automatically. For instance, you can setup a time trigger in Apps Script to email spreadsheets every weekday. Or a trigger to download emails from Gmail to your Google Drive.
Time-based triggers in Apps Script have certain limitations, particularly when it comes to setting up recurring schedules. For example, if you want to create a simple cron job that runs every weekend at around 3 PM, you’d need to set up two separate triggers like this:
function createTimeTrigger() {
ScriptApp.newTrigger('functionName')
.timeBased()
.everyWeeks(1)
.onWeekDay(ScriptApp.Weekday.SUNDAY)
.atHour(15)
.create();
ScriptApp.newTrigger('functionName')
.timeBased()
.everyWeeks(1)
.onWeekDay(ScriptApp.Weekday.SATURDAY)
.atHour(15)
.create();
}
Managing more complex triggers, like one that runs at 10 PM on the 15th of every alternate month, becomes even more challenging. In contrast, writing a cron expression for this is quite straightforward: 0 22 15 */2 *
. Similarly, creating a time trigger that runs at 10:30 am on the last day of every month would involve much more code that writing a cron expression: 30 10 L * *
Google Script meets Cron Expressions
The cron syntax is powerful and supports complicated recurring schedules but, unfortunately, it is not available inside Google Apps Script. But we now have a easy workaround.
We can write our time trigger schedules in cron expressions directly inside Apps Script.
Load the Cron Parser Library
We will use the popular croner
library in Apps Script to parse cron expressions and calculate the upcoming schedules.
const loadCronLibrary = () => {
const key = 'croner@7';
const url = 'https://cdn.jsdelivr.net/npm/croner@7/dist/croner.umd.min.js';
const cache = CacheService.getScriptCache();
// Check if the library content is already cached
const cachedContent = cache.get(key);
if (cachedContent) return cachedContent;
// Fetch the library content from the specified URL
const libraryContent = UrlFetchApp.fetch(url, {
muteHttpExceptions: false,
}).getContentText();
// Check if the fetched content contains the word "Cron"
if (/Cron/.test(libraryContent)) {
// Cache the libary for 6 hours
cache.put(key, libraryContent, 60 * 60 * 6);
return libraryContent;
}
throw new Error('The cron library is unavailable');
};
Add Trigger with Cron Expression
Next, we’ll create a function that loads the Cron library and checks if a scheduled task is set to execute within the next 5 minutes. It uses the script’s timezone to compare the dates.
const scheduledFunction = () => {
// Run the trigger at 3:45 for the 1st 10 days of every month
const cronExpression = '45 3 1-10 * *';
eval(loadCronLibrary());
const job = Cron(cronExpression);
const timeDifference = (job.nextRun() - new Date()) / (1000 * 60);
if (Math.abs(timeDifference) <= 5) {
Logger.log('Hello, I am running via the time trigger!');
}
};
const addTrigger = () => {
ScriptApp.newTrigger('scheduledFunction').timeBased().everyMinutes(5).create();
};
The addTrigger
function would create the time trigger that would invoke the scheduledFunction
every 5 minutes. The cron schedule is checked every 5 minutes, and if it is scheduled to run, the Hello
message would be logged to the console.
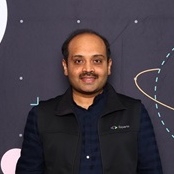
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory