How to Delete Blank Rows from Tables in your Google Documents
How to remove all blank rows from one or more tables in a Google Docs document with Google Apps Script. You may also delete blank rows from tables in Google Slides.
The Document Studio add-on helps you generate Google Documents from data in Google Sheets and Google Form responses. You can create a template in Google Docs and the add-on will replace the placeholders with answers submitted in the Google Form response.
This approach may however create a lot of blank rows in the table for answers that have no response in Google Forms. To give you an example, if the user has not answered the Age
question, the generated document will have a row for the {{Age}}
question but with a blank value.
Remove Blank Rows in Google Docs
With the help of Google Apps Script, we can easily pull all tables that are contained in the body of a Google Document, iterate through each row in the table and, if there’s no value in the row, we can safely remove the row from the table.
Inside your Google Document, go to the Tools menu, choose Script Editor and paste the following code. Go to the Run menu and choose RemoveBlankRows from the dropdown to run the script.
const removeBlankRows = () => {
// Replace all whitespaces and check if the cell is blank
const isBlankCell = (text = '') => !text.replace(/\s/g, '');
// Does the row have any data other than in column 1 (header)
const rowContainsData = (row) => {
const columnCount = row.getNumCells();
let rowHasFilledCell = false;
for (let columnIndex = 1; columnIndex < columnCount && !rowHasFilledCell; columnIndex += 1) {
const cellValue = row.getCell(columnIndex).getText();
if (!isBlankCell(cellValue)) {
rowHasFilledCell = true;
}
}
return rowHasFilledCell;
};
// Get the current document
const document = DocumentApp.getActiveDocument();
document
.getBody()
.getTables()
.forEach((table) => {
const rowCount = table.getNumRows();
for (let rowIndex = rowCount - 1; rowIndex >= 0; rowIndex -= 1) {
const row = table.getRow(rowIndex);
if (isBlankCell(row.getText()) || !rowContainsData(row)) {
// Remove the row from the Google Docs table
table.removeRow(rowIndex);
}
}
});
// Flush and apply the changes
document.saveAndClose();
};
Delete Blank Table Rows in Google Slides
You can use the same technique to remove blank rows from tables that are contained in your Google Slide presentation.
If your Google Slides table uses merged cells, you may want to check merge status of a cell with the SlidesApp.CellMergeState.MERGED
enum.
const removeBlankRows = () => {
// Get the current document
const presentation = SlidesApp.getActivePresentation();
presentation.getSlides().forEach((slide) => {
slide.getTables().forEach((table) => {
const rowCount = table.getNumRows();
for (let rowIndex = rowCount - 1; rowIndex >= 0; rowIndex -= 1) {
const row = table.getRow(rowIndex);
const cellCount = row.getNumCells();
let rowHasFilledCell = false;
for (let cellIndex = 1; cellIndex < cellCount && !rowHasFilledCell; cellIndex += 1) {
const cellValue = row.getCell(cellIndex).getText().asString();
if (cellValue.trim() !== '') {
rowHasFilledCell = true;
}
}
if (!rowHasFilledCell) {
row.remove();
}
}
});
});
// Flush and apply the changes
presentation.saveAndClose();
};
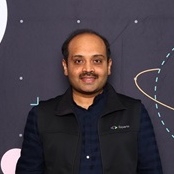
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory