Convert and Email Google Spreadsheets as PDF Files
You can set up a cron job in Google Drive using Google Apps Script that will send any Google Spreadsheet, or any other document or file in the Drive, to one or more email addresses at a specified time. You can set the time-based trigger to run weekly, daily, every hour and other recurring schedules.
This example shows how to send a Google Spreadsheet to the specified email address automatically as a PDF file daily. The Google Script converts the Google Spreadsheet into a PDF file and sends it to another email address using your own Gmail account. You can further customize the PDF output - like remove gridlines, hide frozen rows, change to landscape mode, etc. by setting the correct export parameters.
Convert & Email Google Sheets
The Email Google Spreadsheet add-on can automatically convert and email spreadsheets in PDF, CSV or Microsoft Excel (xlsx) formats. It can convert the entire spreadsheet or individual sheets.
The premium version of the add-on can automatically email the converted sheets on a recurring schedule (like every hour, daily, weekly or monthly). You can also set up multiple sending schedules and automatically email the sheet to a different set of receipts at different times.
Google Script to Email Google Spreadsheets
If you cannot use the Email Spreadsheet add-on (some Google Apps admins do not allow add-ons), you can write your own Google Script to email the spreadsheet as PDF files.
/* Send Spreadsheet in an email as PDF, automatically */
function emailSpreadsheetAsPDF() {
// Send the PDF of the spreadsheet to this email address
const email = Session.getActiveUser().getEmail() || 'amit@labnol.org';
// Get the currently active spreadsheet URL (link)
// Or use SpreadsheetApp.openByUrl("<>");
const ss = SpreadsheetApp.getActiveSpreadsheet();
// Subject of email message
const subject = `PDF generated from spreadsheet ${ss.getName()}`;
// Email Body can be HTML too with your logo image - see ctrlq.org/html-mail
const body = 'Sent with [Email Google Sheets](https://www.labnol.org/email-sheet)';
// Base URL
const url = 'https://docs.google.com/spreadsheets/d/SS_ID/export?'.replace('SS_ID', ss.getId());
const exportOptions =
'exportFormat=pdf&format=pdf' + // export as pdf / csv / xls / xlsx
'&size=letter' + // paper size legal / letter / A4
'&portrait=false' + // orientation, false for landscape
'&fitw=true&source=labnol' + // fit to page width, false for actual size
'&sheetnames=false&printtitle=false' + // hide optional headers and footers
'&pagenumbers=false&gridlines=false' + // hide page numbers and gridlines
'&fzr=false' + // do not repeat row headers (frozen rows) on each page
'&gid='; // the sheet's Id
const token = ScriptApp.getOAuthToken();
const sheets = ss.getSheets();
// make an empty array to hold your fetched blobs
const blobs = [];
for (let i = 0; i < sheets.length; i += 1) {
// Convert individual worksheets to PDF
const response = UrlFetchApp.fetch(url + exportOptions + sheets[i].getSheetId(), {
headers: {
Authorization: `Bearer ${token}`,
},
});
// convert the response to a blob and store in our array
blobs[i] = response.getBlob().setName(`${sheets[i].getName()}.pdf`);
}
// create new blob that is a zip file containing our blob array
const zipBlob = Utilities.zip(blobs).setName(`${ss.getName()}.zip`);
// optional: save the file to the root folder of Google Drive
DriveApp.createFile(zipBlob);
// Define the scope
Logger.log(`Storage Space used: ${DriveApp.getStorageUsed()}`);
// If allowed to send emails, send the email with the PDF attachment
if (MailApp.getRemainingDailyQuota() > 0)
GmailApp.sendEmail(email, subject, body, {
htmlBody: body,
attachments: [zipBlob],
});
}
The Google Script function will convert each of the worksheets of a Google spreadsheet into a PDF file, compresses all the PDF files in a ZIP file and sends it to a designated email address. You can send to multiple email addresses too - just separate each of them with a comma.
The method currently sends all the sheets of a Spreadsheet in the ZIP file but you can also specify a sheet ID with the &gid
parameter to email a particular sheet only. For instance, to send the first sheet, you can set the gid=0
and so on.
Convert Full Google Sheet to a PDF File
The above snippet converts individual sheets into separate PDF files but there’s also a way to convert the entire spreadsheet into a single PDF file. In that case, replace guid=
with id=SS_ID
(spreadsheet ID) or perform the conversion using DriveApp as shown here.
Email Google Spreadsheet as PDF
function emailGoogleSpreadsheetAsPDF() {
// Send the PDF of the spreadsheet to this email address
var email = 'amit@labnol.org';
// Get the currently active spreadsheet URL (link)
var ss = SpreadsheetApp.getActiveSpreadsheet();
// Subject of email message
var subject = 'PDF generated from spreadsheet ' + ss.getName();
// Email Body can be HTML too
var body =
"Sent via <a href='https://workspace.google.com/marketplace/app/email_spreadsheets/431723916752'>Email Spreadsheets</a>";
var blob = DriveApp.getFileById(ss.getId()).getAs('application/pdf');
blob.setName(ss.getName() + '.pdf');
// If allowed to send emails, send the email with the PDF attachment
if (MailApp.getRemainingDailyQuota() > 0)
GmailApp.sendEmail(email, subject, body, {
htmlBody: body,
attachments: [blob],
});
}
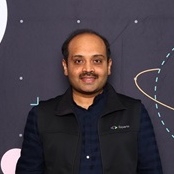
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory