How to Extract Images from Google Docs and Google Slides
Learn how extract all the embedded images from a Google Document or Google Slides presentation and save them as individual files in a specified folder in your Google Drive.
Imagine you’re working with a lengthy Google Document, or a Google Slides presentation, and you need to extract all the embedded images from the text and save them as individual files.
Extract Individual Images
A simple solution to address this issue is as follows: convert your Google Document or Google Slide into a web page. Here’s how you can do it:
Go to the “File” menu. Select the “Share” submenu and then choose “Publish to Web.” It will generate a public web page that contains all the images from your document or slide. You can simply right-click an image on the page and select the “Save Image” option download it to your local disk.
What we have just discussed is a manual process but we can easily automate this with the help of Google Apps Script.
Extract all Images from a Google Document
Open your Google Document containing the images, go to the Extensions menu and choose Apps Script. Copy-paste the code below and run the saveGoogleDocsImages
function to download all images to a specific folder in your Google Drive.
The images are sequentially numbered and the file extension is the same as that of the embedded inline image.
function saveGoogleDocsImages() {
// Define the folder name where the extracted images will be saved
const folderName = 'Document Images';
// Check if a folder with the specified name already exists
const folders = DriveApp.getFoldersByName(folderName);
// If the folder exists, use it; otherwise, create a new folder
const folder = folders.hasNext() ? folders.next() : DriveApp.createFolder(folderName);
// Get all the images in the document's body and loop through each image
DocumentApp.getActiveDocument()
.getBody()
.getImages()
.forEach((image, index) => {
// Get the image data as a Blob
const blob = image.getBlob();
// Extract the file extension from the Blob's content type (e.g., 'jpeg', 'png')
const [, fileExtension] = blob.getContentType().split('/');
// Generate a unique file name for each image based on its position in the document
const fileName = `Image #${index + 1}.${fileExtension}`;
// Set the Blob's name to the generated file name
blob.setName(fileName);
// Create a new file in the specified folder with the image data
folder.createFile(blob);
// Log a message indicating that the image has been saved
Logger.log(`Saved ${fileName}`);
});
}
Extract all Images from Google Slides
The Apps Script code to download images from a Google Slides presentation is similar. The function iterates over the slides in the presentation and then for each slide, the function iterates over the images in that slide.
function extractImagesFromSlides() {
// Define the folder name where the extracted images will be saved
const folderName = 'Presentation Images';
// Check if a folder with the specified name already exists
const folders = DriveApp.getFoldersByName(folderName);
// If the folder exists, use it; otherwise, create a new folder
const folder = folders.hasNext() ? folders.next() : DriveApp.createFolder(folderName);
// Iterate through each slide in the active presentation
SlidesApp.getActivePresentation()
.getSlides()
.forEach((slide, slideNumber) => {
// Retrieve all images on the current slide
slide.getImages().forEach((image, index) => {
// Get the image data as a Blob
const blob = image.getBlob();
// Extract the file extension from the Blob's content type (e.g., 'jpeg', 'png')
const fileExtension = blob.getContentType().split('/')[1];
const fileName = `Slide${slideNumber + 1}_Image${index + 1}.${fileExtension}`;
// Set the Blob's name to the generated file name
blob.setName(fileName);
// Create a new file in the specified folder with the image data
folder.createFile(blob);
Logger.log(`Saved ${fileName}`);
});
});
}
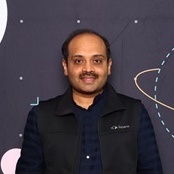
Amit Agarwal
Google Developer Expert, Google Cloud Champion
Amit Agarwal is a Google Developer Expert in Google Workspace and Google Apps Script. He holds an engineering degree in Computer Science (I.I.T.) and is the first professional blogger in India.
Amit has developed several popular Google add-ons including Mail Merge for Gmail and Document Studio. Read more on Lifehacker and YourStory